Communication between Microservices
In this article, we will learn about different ways of communication between microservices.
Microservices Architecture
A microservices architecture is one of the distributed systems which contains multiple services. They will send or retrieve data from each other. Each service interacts together using communication protocols.
Two Ways of Microservice Communication
There are two basic and best ways to communicate between microservices.
- Synchronous communication between microservices
- Asynchronous communication between microservices
Microservices Synchronous Communication
The most common type of synchronous communication is an HTTP request. This protocol supports sending a request and receiving a response from another microservice. In this communication, data transfers to an endpoint of a service in a blocking interaction. A service sends a request and waits for a response from another service.
When a request is sent from Microservice-1 to Microservice-2 through HTTP, Microservice-1 is blocked in the interaction until a response is received.
Implementation using HTTP
While communicating between services using HTTP, it needs to use any one of the HTTP libraries like Axios or superagent.
In the implementation below, we are going to use the axios library. To use that, install the Axios npm package using this command.
npm install axios
Here we are creating two separate services- Posts Service and Comments Service. Both services start in different ports.
Posts service - 4000, Comments service- 4001.
In the above implementation, there are 3 API methods for Posts Service.
- GET/posts -> responsible for getting all the posts.
- POST/posts -> responsible for adding a new post.
- POST/post-event -> responsible for receiving messages when new comments are created.
In the above implementation, there are 3 API methods for Comments Service.
- GET/posts/:id/comments -> responsible for getting all the comments on the post.
- POST/posts/:id/comments -> responsible for adding new comments to the post.
- POST/comment-event -> responsible for receiving messages when new comments are created.
Output and Explanation
We will see how the posts and comments services communicate using HTTP.
Whenever a request(POST/posts) in Posts Service calls, the new post is added and makes another request(POST/comment-event) to Comments Service, which displays the PostCreated in the console.
Whenever a POST/posts/:id/comments request in Comments Service calls, the new comment is added to that post and makes another request(POST/post-event) to Posts Service, which displays the CommentCreated in the console.
It is an HTTP way to communicate between services.
Microservices Asynchronous Communication
The most common type of Asynchronous communication is AMQP(Advanced Message Queuing Protocol) request. It is the protocol for transferring data between microservices through non-blocking interactions via Message brokers.
When a request is sent from Microservice-1 to Microservice-2 through AMQP. A message broker acts as an intermediate that helps to communicate between microservices. It maintains messages until received by Microservice-2.
There are many Messaging brokers available for asynchronous communication.
- RabbitMQ
- Apache Kafka
- Amazon Web Services
- ActiveMQ
- Azure Service Bus
- Google Pub/Sub
In this asynchronous communication, We are going to use the popular message broker - RabbitMQ
RabbitMQ is an open-source message broker software. It processes multiple messages per second. It is lightweight and performs very fast compared to synchronous communication.
RabbitMQ Architecture
There are 4 different parts in the setup of RabbitMQ :
1. Producer - Responsible for sending messages.
2. Exchange - Responsible for sending messages to the queue.
3. Queue - Responsible for storing messages.
4. Consumer - Responsible for receiving and processing messages.
Implementation using Message broker(RabbitMQ)
Before implementation, install the RabbitMQ server using this documentation in your system.
To add RabbitMQ to your NodeJS application, install the amqplib npm package using this command.
npm install amplib
As with the same example, create two separate services- Posts Service and Comments Service. Both services start in different ports.
Posts service - 4000, Comments service- 4001.
- For both the services, first import amplib package import amqp from 'amqplib/callback_api';
- Connect amqp to the rabbitmq server using the connection URL. Here, we are using a local server, so the connection URL is amqp://localhost
- To send or receive messages from a queue, we have to use the createChannel() method for channel creation.
- To communicate between services, we need to create a queue using the assertQueue() method in channels.
- Based on that queue, we can send and receive messages between services.
- To send a message, use the sendToQueue() method along with the queue name and message in string format.
- To receive a message, use the consume() method with the queue name.
- Once we consume the message, we need to acknowledge the message using the ack() method to remove the message from the queue.
In above implementation, there are 2 API methods and 1 consumer for posts.
- GET/posts -> responsible for getting all the posts.
- POST/posts -> responsible for adding new posts.
- Consume/post-event -> responsible for consuming messages from another service.
In the above implementation, there are 2 API methods and 1 consumer for comments.
- GET/posts/:id/comments -> responsible for getting all the comments.
- POST/posts/:id/comments -> responsible for adding new comments.
- Consume/comment-event -> responsible for consuming messages from another service.
Output and Explanation
We will see how the posts and comments services communicate using RabbitMQ.
Whenever a request(POST/posts) in Posts Service calls, the new post is added and sends the message, using the sendToQueue() method with queue name(comment-event) to Comments Service, which consumes the message and displays the PostCreated in the console. Finally, acknowledge the message to the queue.
Whenever a request(POST/posts/:id/comments) in Comments Service calls, the new comment is added to that post and sends the event message using the sendToQueue() method with queue name(post-event) to Posts Service, which consumes the message and displays the CommentCreated in the console. Finally, acknowledge the message to the queue.
It is the way to communicate between services using RabbitMQ.
When we handle multiple requests between microservices using HTTP, it takes more time to receive responses and reduces app performance.
At the same time, Synchronous Communication is not apt for multiple requests.
So Asynchronous Communication is the best way for our app performance and consumes lesser time for execution.
Conclusion
We hope this blog will help you understand the concept of communication between microservices and help you choose the best communication type.
If you are planning to hire Nodejs developers for your project or need any help related to this article topic, connect with our developers!
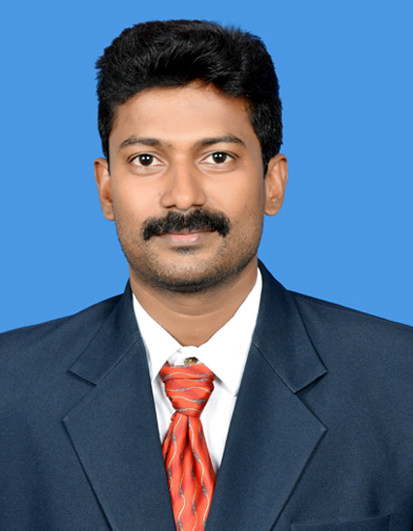
Suresh
I am a Full Stack Developer with over a year of experience, specializing in React, NodeJS, PostgreSQL, and MongoDB. Quick and smart learner is my best attribute.