Node-Cache with Express Node JS
The main objective of this article is to learn the Data Caching concepts in NodeJS.
Caching in Nodejs
In general, caching is a storage mechanism that acts as a secondary storage layer. It helps to increase the server response time.
The significance of Caching is apt for reading the same data several times whereas processed one time.
Also, it helps to improve the performance of the application.
Why is Cache Needed?
The caching enhances the speed of fetching data, especially to fetch data from an external API.
Data once stored in the cache can be retrieved later directly from the cache again until the data is updated.
It does not need to retrieve data from the database every time. Thereby caching makes clients' requests/responses fast.
Let’s see the node-cache concept.
Introduction of node Cache
Node-Cache is a simple and fast internal caching module in NodeJS. It can store data in the app process memory when the data is retrieved from the database for a particular request.
Based on the requests from clients, the data will be sent by cache memory (Process Memory) and not from the database. The Node-Cache package saves the response time in NodeJS.
Node-cache module provides set, get, and deletes methods for data processing.
We will discuss set, get and delete methods later in the implementation of the Node cache in our app.
Advantages of Node Cache
-
Application performance is adequate.
-
Data processing time is less.
-
A minimal requirement for the implementation.
Implementation of Node Cache
To use Node Cache, we need to install the node-cache npm package in our application with the below-mentioned command.
npm install node-cache
In the implementation below, we use a Dummy API record from dummyjson.com instead of a database record.
Multi-varied uses of the node-cache:
-
.set(key, value, [TTL]) is for setting a value to a specified key in the cache. TTL (Time-to-Live) is also used to expire the key at a particular time (in seconds), which is optional, and it returns.
-
.get(key) is utilized for getting a value from the cache to the specified key. If the key is not found it returns undefined.
- .has(key) is used for checking the data from the cache to the specified key. If the key is found it returns true, else returns false.
-
.del(key) is responsible for deleting the key value from the cache. It returns the number of keys deleted.
-
.keys() is utilized for getting all the keys stored in the cache. It returns the keys.
The expiration time of the cache is unlimited. If we need to expire on a specific time, it initiates the option stdTTL as a key with time (in seconds) as value. 0 is unlimited.
const cache = new NodeCache( { stdTTL: 10 } );
Output and Explanation
In this implementation method, we use an API (GET/products) to get all the product data from that fake API database.
First Request
Whenever a request (GET/products) is made, it will check whether data is available in the cache to that specified key (products) using has (key) method. If it is not available, it will fetch data from the fake API database and store it in the cache using the set (key, value) method.
In the aforementioned output, it takes 1.13s to fetch data from the dummy API database.
Second Request
From the next request onwards, it will check whether data is available in the cache to that specified key(products) using has (key) method. If it's available, it will get the data from the cache directly using the get (key) method.
The above output takes only 7ms to fetch data from the cache.
This approach gives better performance to the application when the same data is required repeatedly.
Conclusion
This article will be instrumental in identifying the usage of the Node Cache module in NodeJS. You can refer to the aforementioned implementation of the Node Cache process and compare the performance of the application using Node cache.
Want to connect with Nodejs developers for your project? Here we are! Hire our Nodejs developers.
Recommended Articles:
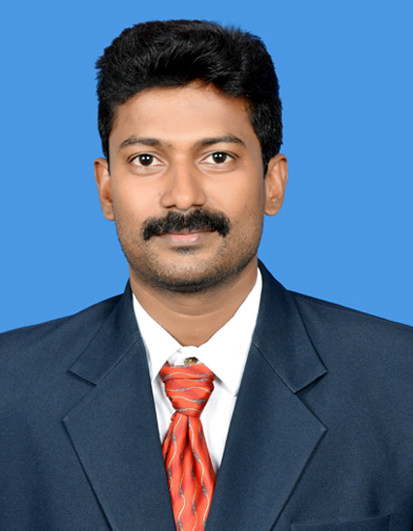
Suresh
I am a Full Stack Developer with over a year of experience, specializing in React, NodeJS, PostgreSQL, and MongoDB. Quick and smart learner is my best attribute.