Optimizing App Performance with Nodejs Cluster
This article aims at the nuances of optimizing NodeJS application performance with clustering.
What is NodeJS Clustering?
NodeJS runs in a single-threaded way. By default, NodeJS is running on a single-core processor for each thread.
In Present times, Every Computing System comes with a multi-core processor. If we are not using the cluster concept, then we are wasting our processor capabilities. Therefore, we should take the positive impacts of all cores to improve our application performance.
For example, Let us take a 4-core machine, where a Node will only be used in a single core. To operate the cluster concept could be used to run 4-core machines. It also helps us to perform 4 times better than a single process. It gives a performance booster to our NodeJS application.
How does the cluster module work?
Cluster creates multiple child processes that run simultaneously with a single parent process (operated parallel by sharing the same server port). Each child process has its V8 instance, event loop, and memory.
There are multiple processes (workers) to handle multiple incoming requests which means several requests can be processed simultaneously and if there is a long-running/blocking operation on one worker, the other workers can continue handling other incoming requests — the app would not wait until the blocking operation completes.
Advantages
⦁ Handle multiple requests to improve application scalability.
⦁ Utilizing all the processors reduce hardware wastage.
⦁ Recreate a new worker can which quickly observe when any child process dies due to heavy load or by any other method.
Disadvantages
⦁ Session management is not possible, alternatives are managed by a developer at the cost of complexity.
How to use Clusters in NodeJS?
To understand the cluster in NodeJS, start with a sample NodeJS app without a cluster.
The above code is a basic nodeJS app with a loop of 10 lakh times. To ensure the performance of the application, let us use the loadtest package
Initially install the loadtest package globally with the command below.
npm install -g loadtest
Then run the app to test with node index.js in the terminal, after opening a new terminal, run the below command appropriately.
loadtest -n 1000 -c 100 http://localhost:5000/withoutCluster
The above command will send 1000 requests to the given target URL, of which 100 are concurrent. Following is the output from running the above command:
The server was able to handle 26 requests per second with a mean latency of 3717.2 milliseconds and a total of 39.0 seconds for 1000 requests.
Further, let us Start the NodeJS app with a cluster module. Before that install cluster package, npm install cluster, and then run the below code
The above code uses a cluster of the module in the nodejs app and operates the same process as before. In this number of child workers are created by using cluster.fork() method based on CPU core size.
Whenever the worker gets kill, the new worker will be created by using cluster.fork() on the “exit” listener.
Thereby, let us run the loadtest for the cluster concept
loadtest -n 1000 -c 100 http://localhost:5000/withCluster
At this moment see, the server was handling 75 requests per second with a mean latency of 1262.1 milliseconds and a total of 13.3 seconds for 1000 requests.
Here without the operation of the cluster, the total time taken to complete 1000 requests was 39.0 seconds, and with the cluster, it only takes 13.3 seconds. Finally, Cluster-based NodeJS application performance is approximately 4 times better than the normal NodeJS application.
Using Process Manager in NodeJS
In this application, the implementation of the cluster concept is manually done by developers to manage workers. When it comes to Production, there is a tool that can help to manage the process called PM2 with a built-in load balancer.
To use PM2, first, you need to install it globally: npm install -g pm2
After PM-2 installation, use the below command, we can access the cluster concept without manual implementation.
pm2 start index.js -i 0
Here, -i denote instance and 0 denotes the maximum number of workers to start an application in cluster mode. You can also use 0 or max to run all the workers.
Conclusion
This operation will enable us to see a greater performance in the improvement of our application which leads to time that handles multiple requests across the worker processes.
Therefore, in the field of production, clustering helps process multiple requests to handle the workload effortlessly.
Want help from Nodejs developers to optimize your app performance? Or want to create node app from scratch?
Talk to our Nodejs experts and hire us for your node js project.
Want to know more about Nodejs?
Recommended Articles
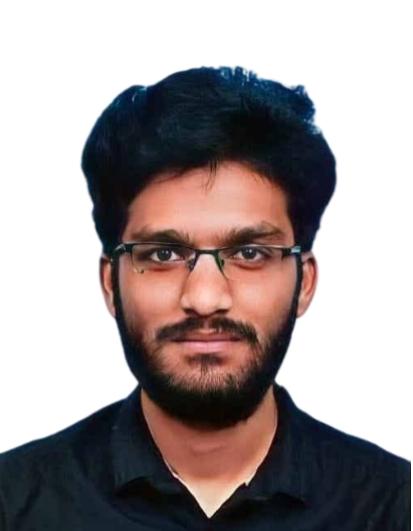
Vasanth
I am a skilled full stack developer with expertise in front-end and back-end development. React, Angular, Vue, NextJS, NuxtJS, Nodejs, DotNet, MySQL, Postgres, SQL Server, MongoDB.