Nodejs Logging – An Ultimate Guide with Example
This blog throws light on resolving the issue of bugs in apps. Imagine that an app is running in a production environment and there are few bugs in an app. We must figure out a way to find out the cause of the bugs and to fix them.
During development time we can use the console.log function to troubleshoot an issue. But this is not recommended for the production.
How might we resolve this issue? The logging solution plays a pivotal role in this situation.
This article will describe the most effective method for writing error logs in Node.js Applications. I will review the value of logging on the other hand to facilitate faster the troubleshooting in Node.js servers.
Introduction to Logging
Building robust web apps requires logging practice as a vital part. Excellent logging approaches are essential for monitoring our Node.js servers.
It aids the developers in quick identification and resolving a variety of application-related to errors and problems.
The process of logging involves creating a message when an event occurs and storing it with the event details in the log store. A log store can be a flat file, database, etc.
Why Nodejs Logging?
Let’s discuss why logging is necessary.
-
If you incorporate a logging solution from the beginning of the project, troubleshooting and fixing issues would be easier.
-
Logging enables developers to quickly identify application issues.
-
We may determine the root cause for the issues fast and provide the apt solutions.
-
Whenever there is an issue with the application, we can gather logs saved in a log store, which makes it simpler to identify the source of issues and handle it.
A few libraries provide logging solutions. The most familiar logging libraries for Node.js are listed below
For the rest of the article, I will use the Winston logging library to demonstrate the various concepts of logging.
Winston
Winston is one of the most popular and straightforward NodeJS logging libraries. This library analyses the app activities and produces information that can be stored in a database or log file. This makes it simpler for developers to store logs. You can quickly set up the storage medium wherever you want to store the logs.
"A logger for just about everything" - Winston.
It allows for universal logging on many transports at different logging levels. It supports Custom logging levels and flexible log formats.
Generally, the key values of Winston are
-
Easy to use and customizable
-
Provides different logging transports, levels, and formats
-
Error monitoring
-
Estimating the duration of app execution
Now, let’s start with the demonstration. We need to install the Winston library to our project to start using logging and use the below command to install the Winston library.
npm install winston express-winston
The above command will install two libraries. One is Winston and another is the dependency for the node express library.
The sample code for Winston using Express is shown below.
-
At first, import the winston and express-winston modules.
logger.js
-
Using the createLogger () method, configure the logger with preferred transports and format.
Initially, let’s discuss the transport property, Winston needs at least one transport to make log files but, in this example, we use multiple transports.
The Winston logger transport property is log storage for our messages. It is used to save generated log messages to a log file.
Using the transport.File () method, we can create a level and filename objects. The logging library gives various names to levels. A unique integer priority is assigned to each level.
Priorities of log levels from 0 to 6 (highest to lowest):
The log levels listed above will be utilized until you define Winston's levels explicitly.
The maximum number of messages that a transport should log can be defined using Winston's level parameter on each transport.
When we get a response for an API call and if the response status code matches with any of the logging levels, then default and custom messages will be stored in the log files as configured.
In the above-mentioned code, we have used three different levels and log files. They are the filename property, which is used to create a log file, the logs that fall below levels of errors will be maintained in the log files (loginformation.log, logwarning.log, etc..).
-
Format is an individual module from Winston using this property, you can access default format methods and styles in your transport.
Examples:
format.json(), format.timestamp(),format.prettyprint() etc..
index.js
In this above code, We have given a custom message in three different levels (info, warn, error) using the logger instance.
-
Let's call the first API with the URL http://localhost:4000/ - the server will send a status code 200 Ok and a Log message (“This is an info message”). Therefore, transport captures custom and default log messages and records them in our log file (logInformations.log).
Output (Log file - logInformations.log ):
- Let’s call the API http://localhost:4000/product - the server will send a status code 400 Bad request and a Log message (“This is a warning message”). And transport captures custom and default log messages and records them in our log file (logWarnings.log).
Output (Log file - logWarnings.log ):
-
Let’s call the API http://localhost:4000/product/detail - the server will send a status code 500 internal server error and a Log message (“This is an error message”). Transport captures custom and default log messages and records them in our log file (logErrors.log).
Output (Log file - logErrors.log ):
Conclusion
These descriptions, coding, and sample logs are all helpful to learn about logging and the Winston Logging Library.
Thereby, you can analyze, troubleshoot, and keep an eye on your app. This reassures the fact that we can save error logs for the future reference.
If you are in the process of developing Nodejs application and need nodejs developers, hire us.
Our Nodejs developers will work on your project to develop an error-free application.
Hire our nodejs developers now!
Recommended Articles:
1) Node-Cache with Express Node JS
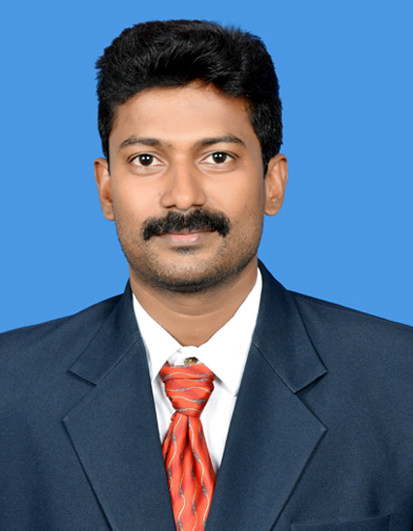
Suresh
I am a Full Stack Developer with over a year of experience, specializing in React, NodeJS, PostgreSQL, and MongoDB. Quick and smart learner is my best attribute.