Redux in React – A Complete Guide
Herein, let’s look at some fundamental principles of Redux and the demonstration of how to use Redux in React. Followed by constructing a simple app using Smart Redux Hooks for improved and simplified understanding.
What is Redux?
Redux is an open-source and client-side State Management library that creates a global store in which the state can be shared between the components without the necessity of having a parent-child connection.
Before proceeding further with Redux, let’s know about State.
What is State?
The State is a plain javascript object that is used to store the data of the component. Whenever the state changes, the component will be re-rendered.
const state = {name: 'react',version: '18'}
When to use Redux?
-
The state is sent across as a prop, to more than two components or hierarchies of the components.
-
The same state is being used by the components without a parent-child connection.
-
Caching page state. For example - when the user visits another page after modifying the form page and returning to the same form page is expected to work in the same condition.
-
When the state changes, the components related to the state can be modified simultaneously.
Redux Pattern
Three components of Redux are:
-
Action
-
Reducer
-
Store
Action
Action is a simple function that returns an object and is responsible for dispatching the data to the store via dispatch function and reducers.
It has two attributes - payload and type. The action is known as the "type" and the value applied to change the state of the application is known as the "payload".
const add = (value) => { dispatch({ type: 'ADD', payload: value, })};
Reducer
The Reducer is a function that changes the state based on certain action types. It takes two arguments - current state and action, and returns a new state. The primary work of a reducer is to create a new state for the store.
const calculatorReducer = (state = 0, action) => {switch(action.type) {case 'ADD': return state + action.payload; case 'SUBTRACT': return state - action.payload; default:return state;}}
Store
The Store is a javascript object and a centralized container to manage the state, accessible to the whole application.
Dispatching the action is the only option to access the store.
{ dispatch: (action) => Dispatch getState: () => State subscribe: (listener: () => void) => () => void}
-
dispatch(action):- Dispatches an action
-
getState():- Returns the current state
-
subscribe(listener):- Respond to the change listener.
Redux Toolkit
By abstracting the Redux API, Redux ToolKit offers various choices for configuring the global store and producing more simplified actions and reducers.
Redux Took Kit uses the following API function, which is an abstraction of the current Redux API function. Redux's flow is not altered by these functions, rather they just simplify them to make them easier to understand and manage.
-
configureStore()
-
createAction()
-
createReducer()
-
createSlice()
-
createAsyncThunk()
-
createEntityAdapter()
The abovementioned APIs can be used to reduce boilerplate code in Redux, particularly the createAction() and createReducer() functions. This may be further simplified by utilising createSlice, which builds action creator and reducer functions automatically.
Demonstration of Redux usage in React
Let’s develop a simple calculator application in React using Redux.
Step 1: Install Dependencies
Create a react app and install the redux libraries: react-redux and @reduxjs/toolkit.
npx create-react-app calculator
cd calculator
npm install react-redux @reduxjs/toolkit
Step 2: Create Actions and Reducers
There are 2 ways to create actions and reducers
-
Traditional way(Separate action and reducer)
-
createSlice function using Redux Toolkit
Let's first examine how reducers and actions appear in traditional React apps.
Create a new file for the reducer function, to change the state value based on the type.
CalculatorAction.js
Create a new file for the reducer function, to change the state value based on the type.
CalculatorReducer.js
Refer above code -
-
The calculatorReducer function takes two arguments state and action.
-
The switch statement is used herein to determine the state based on the action type.
Now let's examine how we may use createSlice to simplify the process and accomplish the same functionality.
Create a new file for the slice, to initialise the state value and reducers to perform actions and change the state value.
As you can see from the above code, all of the actions and reducers are now in one convenient location, as opposed to a typical redux application where each action had to be managed together with its matching action inside the reducer. Using createSlice eliminates the requirement for a switch case to specify the action.
Step 3: Configure Redux Store in Application
Import the following -
-
configureStore from ‘@reduxjs/toolkit’ and
-
Provider from ‘react-redux’
in the top-level component, for connecting the calculatorReducer to the application.
App.js
Refer above code - The configureStore function helps to create a store for the reducers. Provider component has a prop called the store. It contains a Redux store, to access the state of the whole application.
Step 4: Utilize Redux in the component using hooks
Create two components - Calculator and DisplayValue
Calculator.js
Refer above code - This component contains only two buttons, to increment and decrement the value. Here we are using the useDispatch hook which returns a reference of the dispatches function, from the store.
Whenever you click the ‘Increment’ or ‘Decrement’ button, it calls the respective functions (increment() or decrement()) and dispatches the action to the store. Simultaneously, the reducer updates the new state based on the action type.
DisplayValue.js
Refer above code - This is a presentational component and so it displays only the value. Here, the useSelector hook is used which extracts the state from the Redux Store.
Conclusion
In this blog, we examined the various aspects of Redux and the usage of Redux in React. To add more clarity, we developed a simple calculator app that uses the Redux store to update state values and access those values in any interrelated components.
Redux is not necessarily required in all projects and so the concept can be selected based on suitable use cases. Hope this blog helped to understand the Redux concepts and its implementation in React project.
You can hire reactjs developers at Infinijith on a timely basis and we can help you develop your application.
Connect with us to work together!
Recommended Articles:
1) React Hooks- Part 1 (React Basic Hooks)
2) React Hooks- Part 2 (React Additional Hooks)
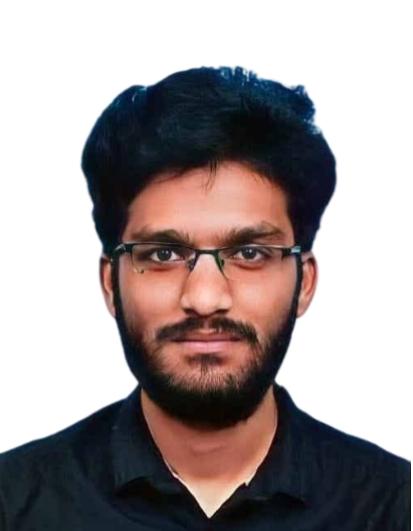
Vasanth
I am a skilled full stack developer with expertise in front-end and back-end development. React, Angular, Vue, NextJS, NuxtJS, Nodejs, DotNet, MySQL, Postgres, SQL Server, MongoDB.